How to Set Up a Blog with Next.js and Sanity: A Comprehensive Guide
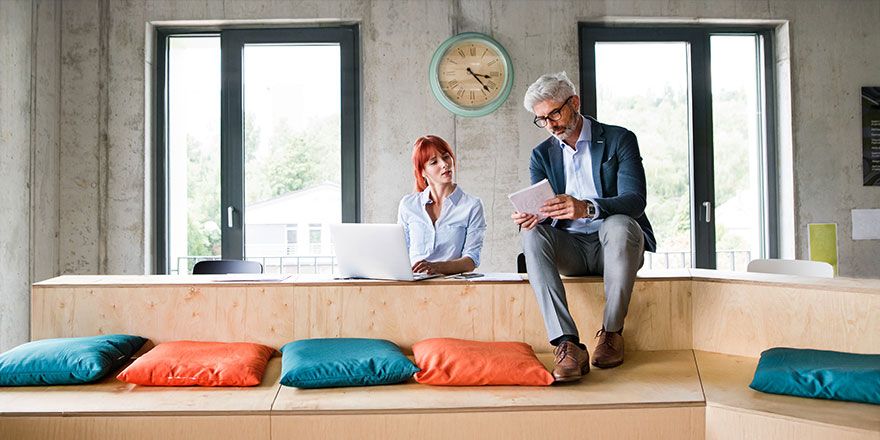
Why Next.js and Sanity?
Next.js provides a great developer experience with features like server-side rendering (SSR), static site generation (SSG), and API routes—all of which can boost performance and SEO. Sanity offers a flexible, real-time CMS with an intuitive interface, allowing you to manage your blog content easily while delivering content through its robust API.
Prerequisites
Before you start, make sure you have:
• Node.js and npm (or yarn): Install from nodejs.org.
• Basic understanding of React and JavaScript.
• A text editor (VS Code is a popular choice).
Step 1: Setting Up a New Next.js App
Let’s begin by creating a new Next.js project. Open your terminal and run:
npx create-next-app my-blog
cd my-blog
This command scaffolds a basic Next.js app for you. Once inside the project directory, you can start the development server:
npm run dev
Visit http://localhost:3000 in your browser to see your fresh Next.js application in action.
Step 2: Creating and Configuring Your Sanity Project
Next, we need to set up Sanity to handle our blog content.
1. Install Sanity CLI:
Open another terminal window and run:
npm install -g @sanity/cli
2. Initialize a new Sanity project:
sanity init --coupon sonysanity2020
During initialization, you’ll be prompted to:
• Choose a project name.
• Select a dataset (usually production).
• Pick a schema template (for a blog, the “Blog” template is a good starting point).
3. Run the Sanity Studio:
Once your project is set up, navigate into your Sanity project folder and run:
sanity start
This opens the Sanity Studio in your browser, where you can add posts, manage content, and adjust your schemas.
Step 3: Connecting Next.js to Sanity
With both your Next.js app and Sanity CMS ready, it’s time to connect them.
1. Install the Sanity Client in your Next.js project:
npm install @sanity/client @portabletext/react
2. Configure the Sanity client:
Create a new file (e.g., lib/sanity.js) in your Next.js project and add the following configuration:
import sanityClient from '@sanity/client';
export const client = sanityClient({
projectId: 'YOUR_PROJECT_ID', // Replace with your project ID
dataset: 'production', // Use your dataset name
useCdn: true, // `false` if you want to ensure fresh data
apiVersion: '2023-03-15', // Use a UTC date string
});
3. Fetching data from Sanity:
In your Next.js pages (e.g., pages/index.js), you can fetch your blog posts with a query. For example:
import { client } from '../lib/sanity';
export async function getStaticProps() {
const query = `*[_type == "post"] { title, slug, body, publishedAt }`;
const posts = await client.fetch(query);
return {
props: { posts },
revalidate: 60, // Re-generate the page every 60 seconds
};
}
export default function Home({ posts }) {
return (
<div>
<h1>My Blog</h1>
<ul>
{posts.map((post) => (
<li key={post.slug.current}>
<a href={`/post/${post.slug.current}`}>
{post.title}
</a>
</li>
))}
</ul>
</div>
);
}
This example uses Next.js’s Static Generation to pre-render your blog homepage, while still keeping your data fresh with revalidation.
Step 4: Building the Blog Pages
With data fetched, the next step is building individual pages for your blog posts.
1. Dynamic Routes for Posts:
Create a dynamic page file under pages/post/[slug].js:
import { client } from '../../lib/sanity';
import { useRouter } from 'next/router';
export async function getStaticPaths() {
const query = `*[_type == "post"]{ slug }`;
const posts = await client.fetch(query);
const paths = posts.map((post) => ({
params: { slug: post.slug.current },
}));
return { paths, fallback: 'blocking' };
}
export async function getStaticProps({ params }) {
const query = `*[_type == "post" && slug.current == $slug][0]`;
const post = await client.fetch(query, { slug: params.slug });
if (!post) {
return { notFound: true };
}
return { props: { post }, revalidate: 60 };
}
export default function Post({ post }) {
return (
<article>
<h1>{post.title}</h1>
<p>{new Date(post.publishedAt).toLocaleDateString()}</p>
<div>
{/* Use a Portable Text renderer if you have complex content */}
{post.body && post.body.map((block, i) => (
<p key={i}>{block.children && block.children[0].text}</p>
))}
</div>
</article>
);
}
2. Styling Your Blog:
Customize your blog by adding CSS or using a framework like Tailwind CSS. For example, install Tailwind CSS following the official guide to enhance your blog’s visual appeal.
Step 5: Deploying Your Blog
Once your blog is set up locally, it’s time to deploy!
1. Deploying Next.js:
Platforms like Vercel offer seamless deployment for Next.js applications. Simply connect your GitHub repository to Vercel, and your blog will be live.
2. Deploying Sanity Studio:
You can deploy Sanity Studio using services like Netlify or Vercel. Follow the Sanity deployment guide for detailed instructions.
Remember to configure environment variables (like your Sanity project ID) on your hosting platforms to ensure smooth connectivity between your Next.js app and Sanity.
Wrapping Up
Congratulations! You’ve now built a dynamic blog using Next.js and Sanity. This setup not only offers a lightning-fast, SEO-friendly front end but also provides a robust content management system behind the scenes.
What’s Next?
• Enhance Your Blog: Add features such as search functionality, comments, or integrations with social media.
• Improve Content Editing: Customize your Sanity schemas to better suit your content needs.
• Optimize Performance: Leverage Next.js image optimization and dynamic imports to further boost performance.
By combining the strengths of Next.js and Sanity, you can build a blog that’s both powerful and flexible, ready to grow as your content evolves.
Happy coding and blogging!