Migrating Your Angular Components to a Signal-Based Approach
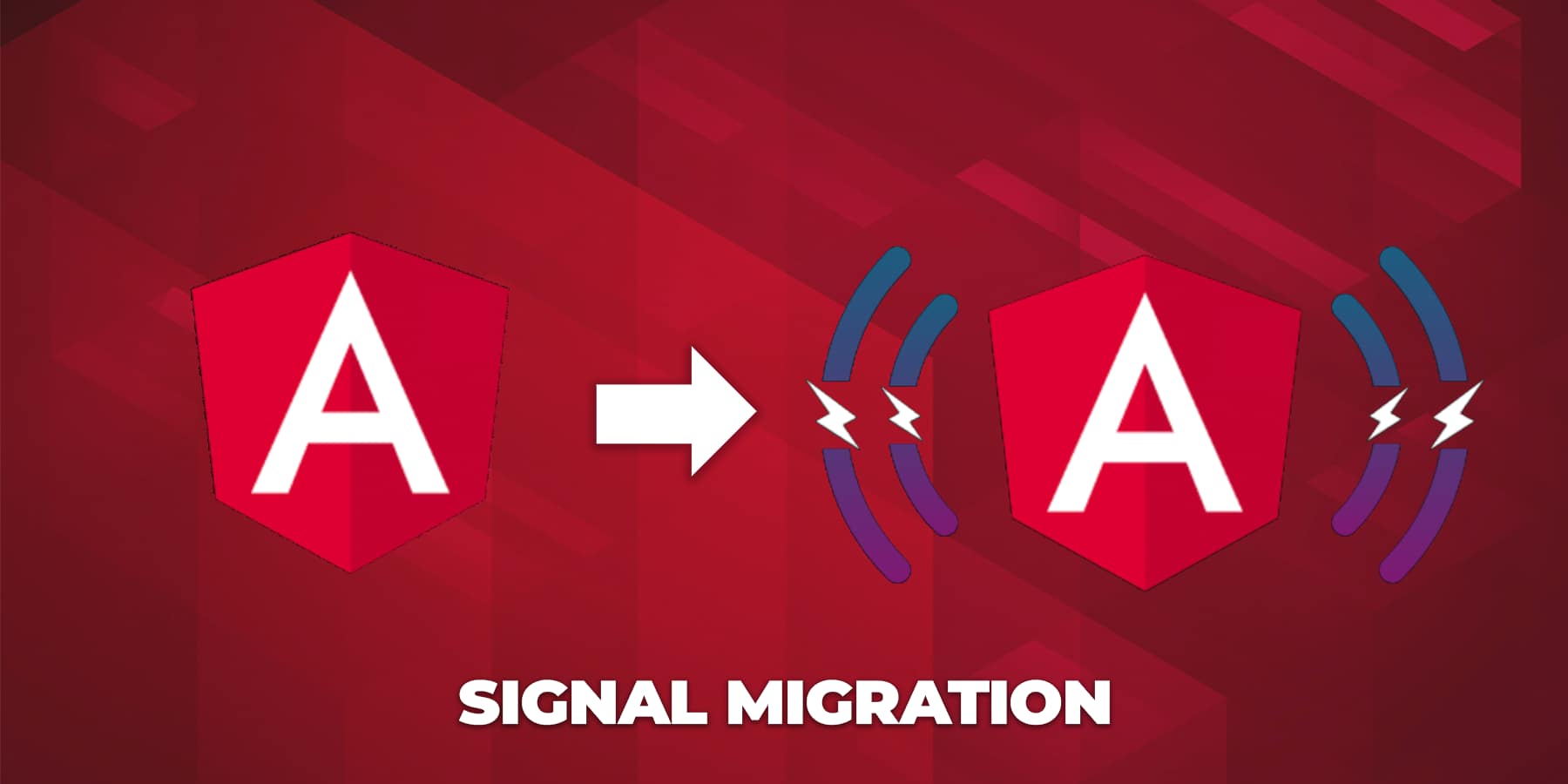
1. Pure Display Component with Input Props
Step 1: Replace the property decorator with the input function.
Step 2: Update your template to access the signal’s value.
Before
import { Component, Input, signal } from '@angular/core';
@Component({
selector: 'app-simple-display',
template: `<div>{{ data() }}</div>`
})
export class SimpleDisplayComponent {
@Input() data: Signal<string> = signal('');
}
After:
import { Component, signal, input } from '@angular/core';
@Component({
selector: 'app-simple-display',
template: `<div>{{ data() }}</div>`
})
export class SimpleDisplayComponent {
data = input<string>('');
}
Explanation: The component now declares its input using the input function. The template remains the same by calling data() to retrieve the current value.
---
2. Component with Output Props
Step 1: Define your output event using the output function.
Step 2: Continue to update any internal state with signals and emit events accordingly.
Before:
import { Component, EventEmitter, Output, signal } from '@angular/core';
@Component({
selector: 'app-event-button',
template: `<button (click)="onClick()">Click me</button>`
})
export class EventButtonComponent {
@Output() clicked = new EventEmitter<void>();
clickCount = signal(0);
onClick() {
this.clickCount.update(count => count + 1);
this.clicked.emit();
}
}
After:
import { Component, signal, output } from '@angular/core';
@Component({
selector: 'app-event-button',
template: `<button (click)="handleClick()">Click me</button>`
})
export class EventButtonComponent {
clickCount = signal(0);
clicked = output<void>();
handleClick() {
this.clickCount.update(count => count + 1);
this.clicked.emit();
}
}
Explanation: Here, the clicked event is now defined using the output function. The rest of the logic remains consistent, ensuring that the component continues to track internal state with signals.
---
3. Component with an API Call
Step 1: Define your data property as a signal.
Step 2: Update the signal once the API call returns data.
Before:
import { Component, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-data-fetch',
template: `<div *ngIf="data">{{ data }}</div>`
})
export class DataFetchComponent implements OnInit {
data: string = '';
constructor(private http: HttpClient) {}
ngOnInit() {
this.http.get<string>('https://api.example.com/data')
.subscribe(result => this.data = result);
}
}
After:
import { Component, OnInit, signal } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-data-fetch',
template: `<div>{{ data() }}</div>`
})
export class DataFetchComponent implements OnInit {
data = signal<string>('');
constructor(private http: HttpClient) {}
ngOnInit() {
this.http.get<string>('https://api.example.com/data')
.subscribe(result => this.data.set(result));
}
}
Explanation: Even though this component doesn’t involve input or output bindings, we use signals to manage the API response data in a reactive way.
---
4. Component Bound to an NgRx Store via Selector
Step 1: Convert the store’s observable into a signal using a helper function (like toSignal).
Step 2: Directly use the signal value in your template.
Before:
import { Component } from '@angular/core';
import { Store, select } from '@ngrx/store';
import { Observable } from 'rxjs';
import { selectFeature } from './store/selectors';
@Component({
selector: 'app-ngrx-binding',
template: `<div *ngIf="data$ | async as data">{{ data }}</div>`
})
export class NgrxBindingComponent {
data$: Observable<string>;
constructor(private store: Store) {
this.data$ = this.store.pipe(select(selectFeature));
}
}
After:
import { Component } from '@angular/core';
import { Store } from '@ngrx/store';
import { toSignal } from '@ngrx/store/signal'; // Hypothetical helper
import { selectFeature } from './store/selectors';
@Component({
selector: 'app-ngrx-binding',
template: `<div>{{ data() }}</div>`
})
export class NgrxBindingComponent {
data = toSignal(this.store.select(selectFeature));
constructor(private store: Store) {}
}
Explanation: Converting the observable to a signal allows for a simplified template binding, making the component code easier to follow.
---
Now that your components are refactored using functional inputs and outputs, it’s time to update your tests as well! You’ll need to adjust your testing strategies to check signal values and output events without relying on traditional decorator-based approaches. Stay tuned for our next post on migrating your unit tests to this new, reactive style.
Happy coding and smooth migrations!